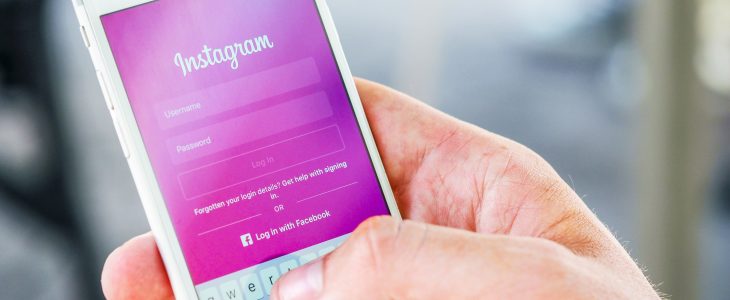
Simple Registration wizard form in PHP, SQL and jQuery
In this example I want to show you simple registration wizard form in PHP, SQL and jQuery. User can choose previous / next button to navigate through form tabs. Our wizard form contains three tabs . First tab is Account next Credentials and the last one – General. Once the registration process is completed all input is stored in database and user will get the message: ” You have registered succesfully!”.
I decided to show you wizard form as they help to make a squel interaction in a more user friendly manner. They are also visually appealing for people. Usually you can see them while signing up into mobile application or during the welcome tour of an application. Another main usuage of wizards app are forms which I decided to introduce today. I have to mention as well that various techniques are used in wizards forms to encourage user to give more personal information. Bootstrap has a great collection of free to use wizards forms- if you are interested to see some of them just click on the link.
In case you would be interested in some wizards plugins I found a nice article for you as well here.
HTML Registration Wizard Form ( full code in download )
<ul id="registration-step"> <li id="account" class="highlight">Account</li> <li id="password">Credentials</li> <li id="general">General</li> </ul> <form name="frmRegistration" id="registration-form" method="post"> <div id="account-field"> <label>Email</label><span id="email-error" class="registration-error"></span> <div><input type="text" name="email" id="email" class="demoInputBox" /></div> </div> <div id="password-field" style="display:none;"> <label>Enter Password</label><span id="password-error" class="registration-error"></span> <div><input type="password" name="password" id="user-password" class="demoInputBox" /></div> <label>Re-enter Password</label><span id="confirm-password-error" class="registration-error"></span> <div><input type="password" name="confirm-password" id="confirm-password" class="demoInputBox" /></div> </div> <div id="general-field" style="display:none;"> <label>Display Name</label> <div><input type="text" name="display-name" id="display-name" class="demoInputBox"/></div> <label>Gender</label> <div> <select name="gender" id="gender" class="demoInputBox"> <option value="female">Female</option> <option value="male">Male</option> </select></div> </div> <div> <input class="btnAction" type="button" name="back" id="back" value="Back" style="display:none;"> <input class="btnAction" type="button" name="next" id="next" value="Next" > <input class="btnAction" type="submit" name="finish" id="finish" value="Finish" style="display:none;"> </div> </form>
Registration Wizard Navigation using jQuery ( full code in dowload )
$(document).ready(function() { $("#next").click(function(){ var output = validate(); if(output) { var current = $(".highlight"); var next = $(".highlight").next("li"); if(next.length>0) { $("#"+current.attr("id")+"-field").hide(); $("#"+next.attr("id")+"-field").show(); $("#back").show(); $("#finish").hide(); $(".highlight").removeClass("highlight"); next.addClass("highlight"); if($(".highlight").attr("id") == $("li").last().attr("id")) { $("#next").hide(); $("#finish").show(); } } } }); $("#back").click(function(){ var current = $(".highlight"); var prev = $(".highlight").prev("li"); if(prev.length>0) { $("#"+current.attr("id")+"-field").hide(); $("#"+prev.attr("id")+"-field").show(); $("#next").show(); $("#finish").hide(); $(".highlight").removeClass("highlight"); prev.addClass("highlight"); if($(".highlight").attr("id") == $("li").first().attr("id")) { $("#back").hide(); } } }); });
PHP MySQL New User Registration
<?php if(count($_POST)>0) { require_once("dbcontroller.php"); $db_handle = new DBController(); $query = "INSERT INTO users (email, password, display_name, gender) VALUES ('" . $_POST["email"] . "', '" . $_POST["password"] . "', '" . $_POST["display-name"] . "', '" . $_POST["gender"] . "')"; $result = $db_handle->insertQuery($query); if(!empty($result)) { $message = "You have registered successfully!"; unset($_POST); } else { $message = "Problem in registration. Try Again!"; } } ?>
Pictures below shows how demo should look like once you install .sql file in your local host. All settings are default ( no password ).
Output of user registration succesfully completed
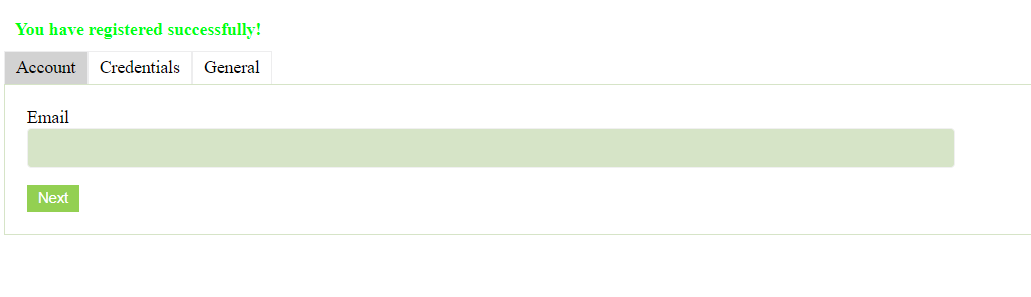
Demo of phpMyAdmin wizard_registration database containing users table
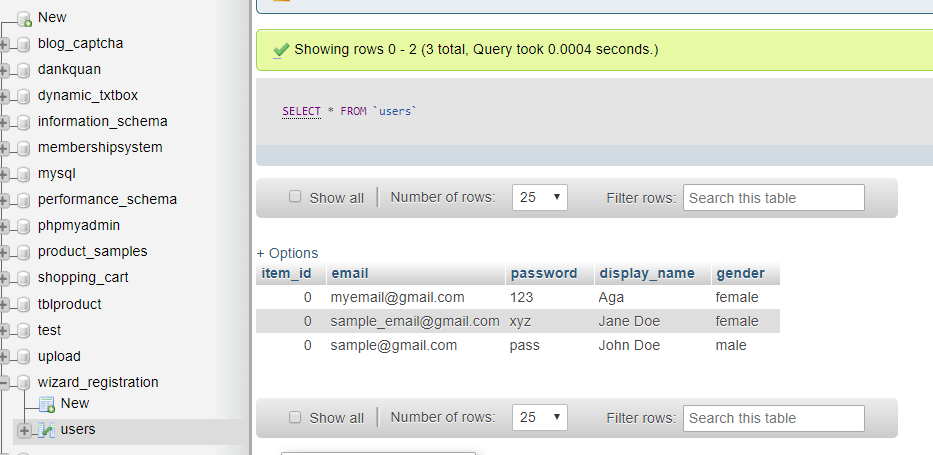
Hope you learned something new
If you learned something new from this tutorial or if you have any question let me know in comments section.